Trace your Python code
with pyftrace.
pyftrace is an open source lightweight Python function tracing tool,
enables fast code analysis and debugging.
Installation #
Simply run:
Basic Tracing #
Example code:
foo
defined in line 1, called in line 8, returns 10bar
defined in line 5, called in line 2, returns 20
foobar.py
1 def foo():
2 bar()
3 return 10
4
5 def bar():
6 return 20
7
8 foo()
Get the calls and returns at a glance with a single line.
Built-in & Path tracing #
pyftrace supports external library function/built-in trace.
builtins.py
1 import os
2
3 def foo():
4 print(os.path.basename(__file__))
5
6 foo()
You will get the following output with following format:
-
Called {function} @ {defined_path}:{line_number} from {called_path}:{line_number}
-
Returning {function} -> {return value} @ {returned_path}
Execution Report #
You can also get the number of calls or duration per function.
fibonacci.py
1 def fibonacci(n):
2 if n <= 1:
3 return n
4 else:
5 return fibonacci(n-1) + fibonacci(n-2)
6
7 result = fibonacci(5)
TUI mode #
pyftrace also provides a Terminal User Interface (TUI) mode.
Analyze large and complex codebases interactively.
PgUp
orPgDn
: Scroll up or down one page at a time.Home
orEnd
: Jump to the beginning or end of the trace.←
or→
: Scroll left or right horizontally.q
: Quit the TUI mode.
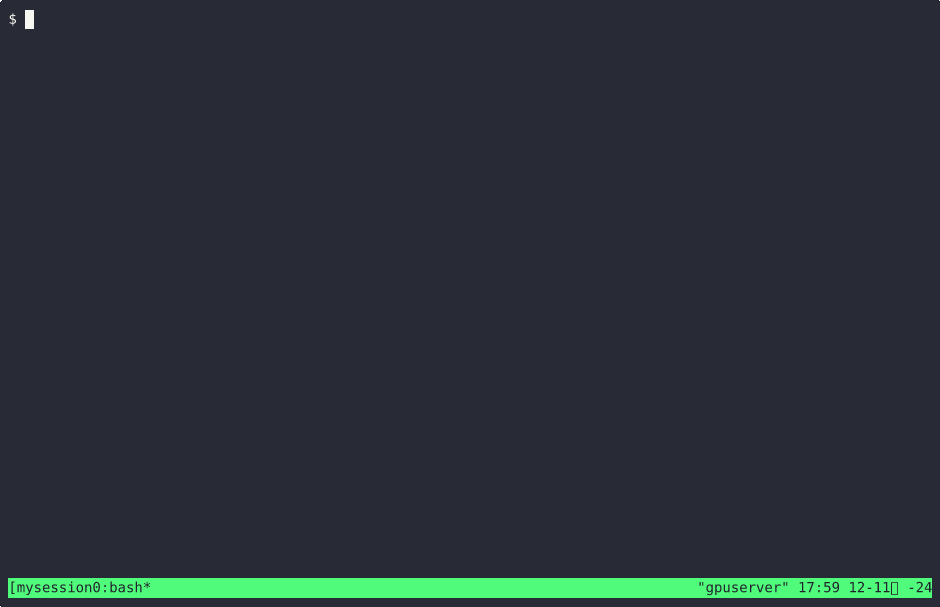
Tool Comparison #
There are other fantastic function tracing tools besides pyftrace (including Traceback inside Python).
But pyftrace has its own advantages:
pyftrace | Traceback | py-spy | viztracer | |
---|---|---|---|---|
Real-time Trace | O | X | O | O |
TUI Mode | O | X | X | X |
Built-in Trace | O | O | O | O |
Execution Report (call count, duration) |
O | X | X | O |
Call-Return hierarchy | O | O | X | O |
Help #
pyftrace was first introduced at Pycon Korea 2024 and is currently maintained by a single maintainer developer.
Your feedback is essential for the project's development.
If you have any feedback, please leave an issue, or at least support pyftrace with a star,
it will be greatly appreciated.
Thanks for reading :)